Loading images from the file system
When you create textures and use them on nodes in your Kanzi Studio project, Kanzi places these texture images into a .kzb binary file. However, you can dynamically load and use in your Kanzi application images from the file system of the device where your Kanzi application is running.
To load images from the file system:
- In Kanzi Studio create a project with C++ application.
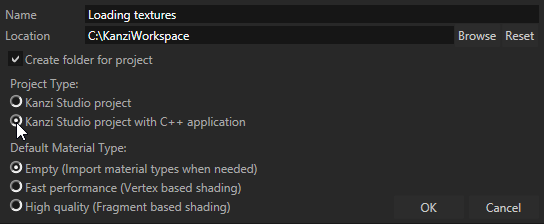
- In the Library right-click Materials and Textures, select Load Material Type From Disk and load a material type that supports textures. See Creating textured materials.
For example, from the <KanziInstallation>/Studio/Asset Library/MaterialTypes load TexturedMaterial or PhongTextured material type. Create a material from the material type. You do not have to assign a texture to this material.
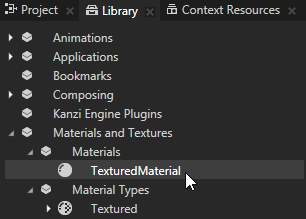
- In the Project create nodes on which you want to dynamically apply textures, add to each object the Texture property, and assign a resource ID to the property.
For example:- Create a Box.
- Add to the Box the Texture property, and assign a resource ID BoxTexture.
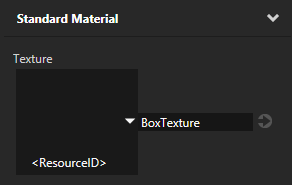
- Set the Material property of the Box to a material that supports textures.
For example, use TexturedMaterial.
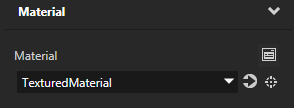
- In the Project press Alt and right-click the node you created in the previous step and select Alias. See Using aliases.
Kanzi Studio adds an alias to the nearest node with a Resource Dictionary. You use aliases in the API to get the objects to which you want to assign textures.
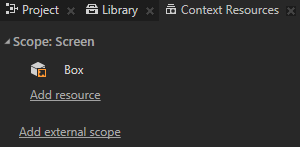
- In Kanzi Studio select > Export KZB > Export KZB Binary.
Kanzi Studio creates the .kzb binary and configuration files from your Kanzi Studio project. Kanzi Studio stores the exported files in <KanziWorkspace>/Projects/<ProjectName>/Application/bin or the location you specify in the Binary Export Directory property in > . The .kzb binary file contains all nodes and resources from your Kanzi Studio project, except the resources you mark in a localization table as locale packs.
When you run your Kanzi application from Visual Studio, your Visual Studio solution reads these files to create your Kanzi application.
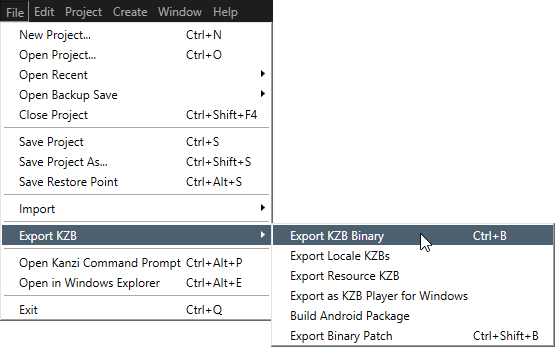
- Place the images you want to use in textures to <ProjectName>/Application/bin.
- In Visual Studio open the Visual Studio solution for your application located in <ProjectName>/Application/configs/platforms/win32. In the C++ application load the textures.
// If you created a Box, iInclude the model3d.hpp header
#include <kanzi/node/model3d.hpp>
...
// In the main class of the application use the onProjectLoaded()
// virtual function to load the file from the file system.
virtual void onProjectLoaded()
{
// Get the Screen of your application
ScreenSharedPtr screenNode = getScreen();
// Get the Box using the #Box alias.
Model3DSharedPtr box = screenNode->lookupNode<Model3D>("#Box");
// Load the texture from the <ProjectName>/Application/bin directory
box->addResource(ResourceID("BoxTexture"), "file:///Red.png");
}
- In Visual Studio select one of the solution configurations for your version of Visual Studio and run your application.
For example, if you are still developing your application, select the GL_vs2010_Debug configuration. If you want to create a production version of your Kanzi application, select one of the available release configurations.
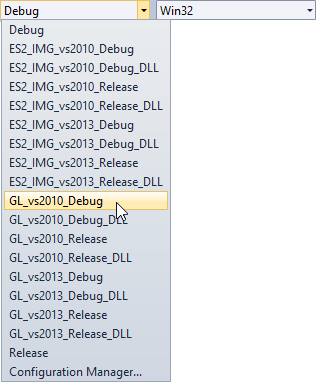
When you launch the application, Kanzi loads the images stored in <KanziWorkspace>/Projects/<ProjectName>/Application/bin and creates textures from the images. When Kanzi loads the application kzb binaries, it sets the textures to the objects you get in the onProjectLoaded
function.
See also
Using .kzb binaries
API reference
Open topic with navigation